Introduction
Welcome to this comprehensive React Installation Guide! If you’re eager to dive into modern frontend development, React is one of the most popular and versatile libraries you can choose. In this post, we’ll walk you through every step required to get React up and running on your machine—whether you’re on Windows, macOS, or Linux.
By the end of this guide, you’ll have a solid understanding of setup methods, tools, and troubleshooting strategies, so you can spend less time configuring environments and more time building dynamic user interfaces.
What is React?
React is an open-source JavaScript library created by Facebook for building declarative, component-based user interfaces. Instead of manually manipulating the DOM, React uses a virtual DOM to batch updates efficiently, resulting in smoother performance and a more predictable development workflow.
Beyond the core library, React boasts an ecosystem of tools—like React Router for navigation and Redux or Zustand for state management—that make scaling applications easier. Its modular architecture encourages reusability and maintainability, which is why React has become a staple in modern web development.
Prerequisites for Installing React
Before diving into installation methods, ensure you have the following prerequisites:
1. Node.js (v14 or higher) including npm or yarn.
2. A modern code editor (e.g., Visual Studio Code).
3. Basic knowledge of JavaScript ES6 features.
Methods of Installing React
There are two primary ways to install React today:
• Create React App (CRA) – The official, zero-configuration CLI.
• Vite – A lightweight, fast bundler for rapid development.
Installing React using Create React App (CRA)
Create React App remains the go-to method for many developers due to its simplicity and opinionated setup. To create a new React project with CRA, run the following command in your terminal:
npx create-react-app my-app
This command downloads the latest CRA template, installs React along with Webpack and Babel configurations, and sets up a local development server. After installation completes, start your app with:
cd my-app
npm start
Your app will be available at http://localhost:3000, displaying the React welcome screen. To customize configurations, you can run `npm run eject`, but be aware that this is irreversible.
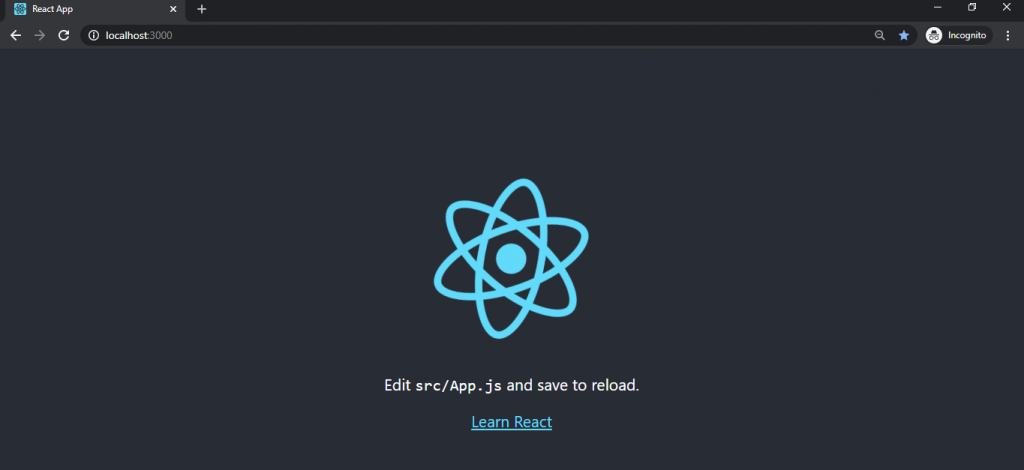
Installing React with Vite
Vite offers a leaner, faster development experience by leveraging native ES modules. To scaffold a Vite-powered React project, use:
npm init vite@latest my-vite-app -- --template react
Then install dependencies and start the development server:
cd my-vite-app
npm install
npm run dev
Your app will be available at http://localhost:5173. Vite’s HMR speeds up development, and for production builds, run `npm run build` to generate optimized assets.
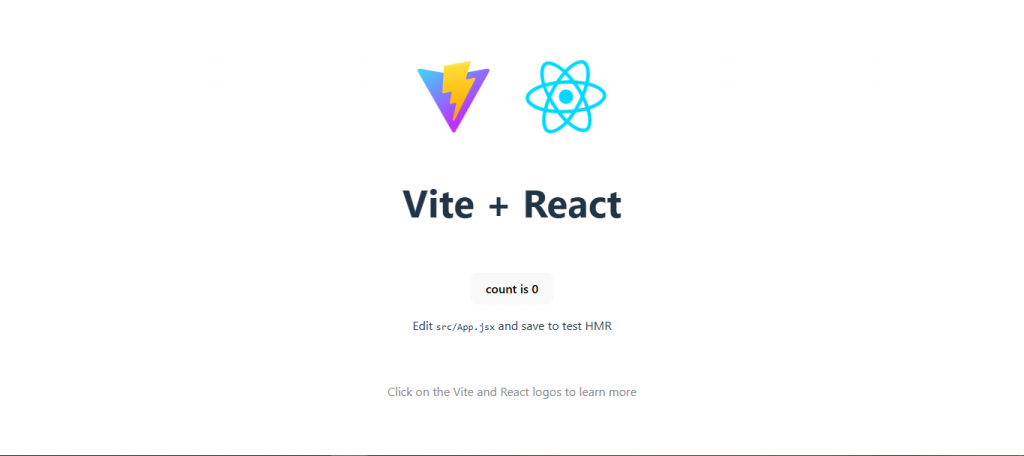
Installing React on Windows, macOS, and Linux
The core React installation steps remain consistent across operating systems, but here are some tips:
• Windows: Use PowerShell or WSL for better compatibility with Unix-style commands.
• macOS: Use Homebrew (`brew install node`) to manage Node.js.
• Linux: Install Node.js via your distro’s package manager (e.g., apt, yum, pacman) or nvm for version management.
Troubleshooting Installation Issues
Here are solutions to common problems you might encounter:
• Permission errors during npm install:
Fix by changing npm directory ownership:
sudo chown -R $(whoami) ~/.npm
• Port conflicts:
Specify a different port:
npm start -- --port 3001
• Incompatible Node versions:
Upgrade using nvm:
nvm install node
nvm use node
Conclusion
You’ve now completed this structured React Installation Guide. Whether you chose Create React App for its zero‑config setup or Vite for its speed, your environment is ready for building interactive, high-performance user interfaces.
FAQ
1. What is the best way to install React?
The best way to install React depends on your project requirements and familiarity with build tools. For newcomers or rapid prototyping, Create React App (CRA) provides a zero‑configuration setup that bundles Webpack, Babel, and a development server out of the box, allowing you to start coding immediately without worrying about toolchain details. However, if you value faster startup times and leaner builds, Vite is an excellent alternative, leveraging native ES modules for near‑instantaneous hot module replacement. Ultimately, choose CRA for stability and convention, and Vite for speed and modern workflow.
2. Do I need Node.js to install React?
Yes, Node.js is essential for installing and managing React because it includes npm (Node Package Manager) and can also support Yarn. These package managers handle downloading React’s core library, its dependencies, and any additional modules you include in your application. Beyond package management, Node.js provides a runtime for development tools such as linters, test runners, and build scripts, making it a cornerstone of the modern JavaScript ecosystem.
3. How do I install React on Windows?
On Windows, you can install React using either PowerShell or the Windows Subsystem for Linux (WSL). First, install Node.js from the official installer or via package managers like Chocolatey. If using WSL, set up a Linux distribution and install Node.js inside that environment to maintain consistency with Unix‑style commands. Once Node.js is ready, navigate to your project folder and run `npx create-react-app my-app` or use Vite with `npm init vite@latest my-vite-app — –template react`.
4. Can I use React without Create React App (CRA)?
Absolutely. For full control over your build process, you can set up React manually with tools like Webpack and Babel, allowing you to tailor configurations for code splitting, asset optimization, and custom plugins. This approach requires more initial setup and maintenance but is ideal for complex projects with specific requirements. Alternatively, other scaffolding tools like Next.js for server‑side rendering or Gatsby for static site generation can provide additional features on top of React.
5. Is Vite better than CRA for setting up React?
Vite generally outperforms CRA in terms of development speed, thanks to its native ES module support and on‑demand compilation, which significantly reduce cold starts and hot update times. However, CRA comes with a battle‑tested configuration that works seamlessly across a wide range of environments and plugins. If you prioritize rapid iteration and minimal configuration, Vite is the better choice; if you need a robust, community‑supported setup with extensive documentation, CRA remains a solid option.
6. Does React work on Linux and macOS?
Yes, React runs on any operating system that supports Node.js, including Linux and macOS. On macOS, you can install Node.js using Homebrew (`brew install node`), while on Linux, you can use your distribution’s package manager or nvm for version management. Once Node.js is installed, the commands for creating and running a React project are identical to those on Windows, ensuring a consistent developer experience across platforms.
7. What additional tools are helpful for React development?
In addition to Node.js and a package manager, consider incorporating Git for version control, ESLint and Prettier for code linting and formatting, and Husky for managing Git hooks. TypeScript can add static type checking to catch errors at compile time, while React Router simplifies navigation in single‑page applications. Testing libraries like Jest and React Testing Library are essential for validating components and behavior, and performance tools like Webpack Bundle Analyzer help optimize bundle size.
Nice walkthrough! One suggestion: adding common pitfalls during the setup—like port conflicts or Node version mismatches—could help beginners avoid some early frustrations.